Prisma – “TypeError: The “payload” argument must be of type object. Received null” Next.js 15 and React 19
Professional Software Developer Since 2010.
Have you also been facing this weird error?
“TypeError: The “payload” argument must be of type object. Received null at … “
Turns out for the case of Prisma + NextJs 15 + React 19 you need in the catch block to specify for it to log specifically “error.stack”
After spending several hours myself, I finally found this comment (opens in new tab)on Reddit.
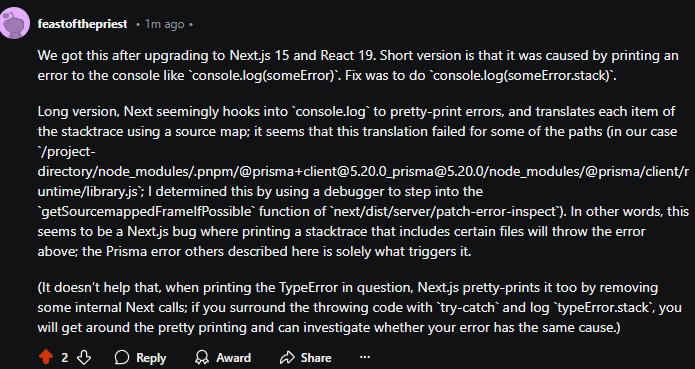
This guy deserves an award for the efforts he put to finally reveal what was the issue for this.
Problem Code Example
To show in example, here are some samples of my code of what I faced:
let interaction;
try {
const contentInteraction: Prisma.ContentInteractionCreateInput = {
contentType: 1,
type,
message,
ipAddress,
userAgent,
userInfo: {
browser: parser.getBrowser().name || "Unknown",
os: parser.getOS().name || "Unknown",
},
}
interaction = await prisma.contentInteraction.create({
data: {
...contentInteraction,
video: {
connect: {
id: id
}
}
},
include: {
video: true,
},
});
} catch (error) {
console.error('Failed to create content interaction:', error);
throw new Error('Failed to record your interaction. Please try again.');
}
Fix Example
And this is simply how I was able to resolve it.
For my case, it turns out I had a unique field constraint that I forgot about, however, working with no debug or error logs, made it almost impossible to find the issue.
let interaction;
try {
const contentInteraction: Prisma.ContentInteractionCreateInput = {
contentType: 1,
type,
message,
ipAddress,
userAgent,
userInfo: {
browser: parser.getBrowser().name || "Unknown",
os: parser.getOS().name || "Unknown",
},
}
interaction = await prisma.contentInteraction.create({
data: {
...contentInteraction,
video: {
connect: {
id: id
}
}
},
include: {
video: true,
},
});
} catch (error) {
if (error instanceof Error) {
console.log(error.stack);
console.error('Failed to create content interaction:', error);
}
console.error('Failed to create content interaction:', error);
throw new Error('Failed to record your interaction. Please try again.');
}
Conclusion
Always wise to wait for new libraries to be tested and mature before we implement in prod right? But if we don’t be first to try then who would? Somebody got to!
Hope this saves your time!
Thanks for reading!